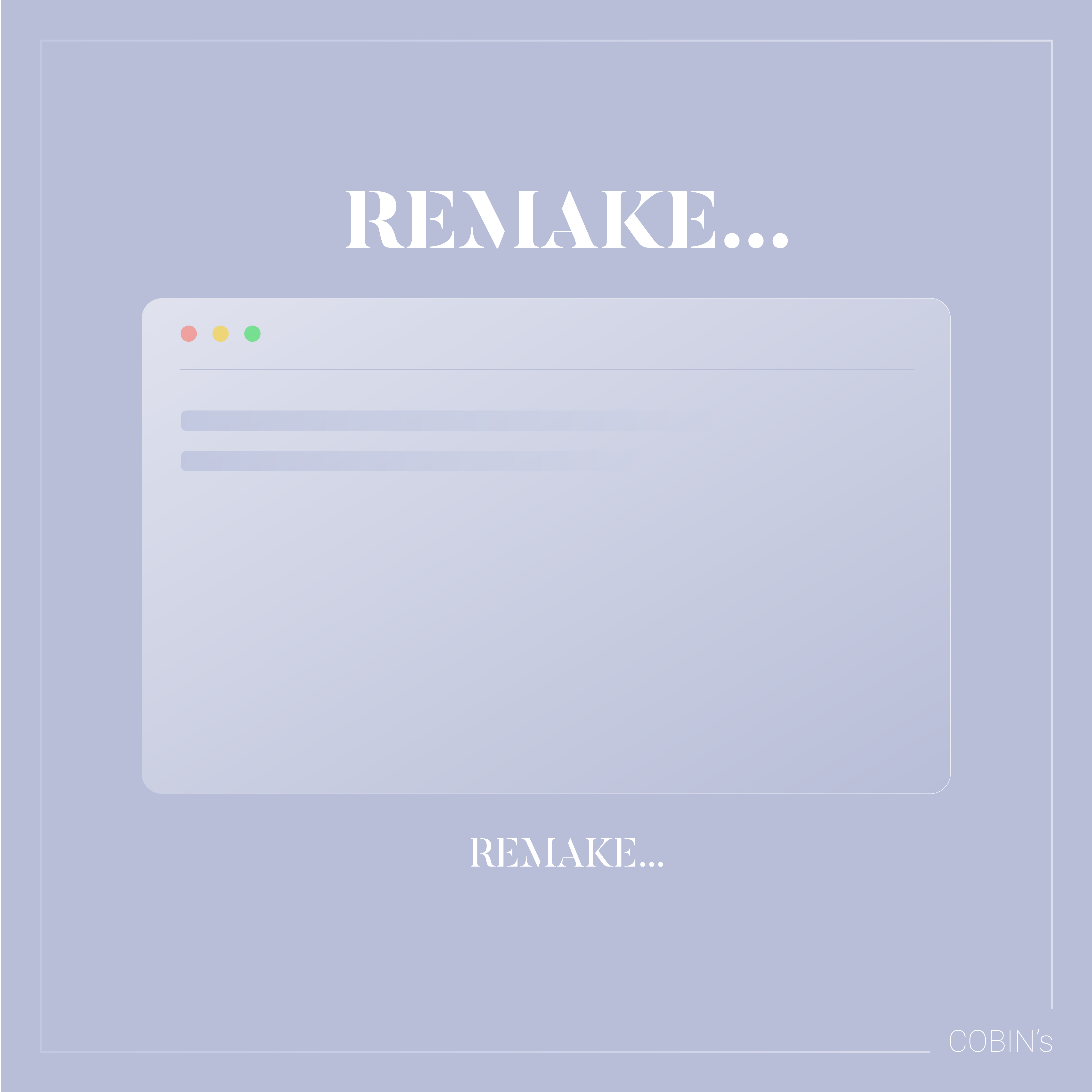
마우스 이펙트 02 (마우스 따라다니기 : GSAP)
이번에는 마우스에 효과를 넣은 이펙트를 코딩해봤습니다.
HTML
HTML은 기본적인 섹션안에 div 클래스를 잡아줍니다.
코드 보기
<main id="main">
<section id="mouseType">
<div class="mouse__cursor"></div>
<div class="mouse__cursor2"></div>
<div class="mouse__wrap">
<p>Money is not everything in the <span class="style1">world,</span> <span class="style2">but the world</span><span class="style3"> runs on money.</span></p>
<p>돈이 <span class="style4">세상에</span> 다는 아니지만<span class="style5">세상은</span> <span class="style6">돈으로</span> 굴러간다.</p>
</div>
</section>
</main>
CSS
css는 기본적인 형태와 스타일을 잡아주지만, 이미지 개개인에 nth-child를 이용해여 개별 구역을 잡아줍니다.
또한, z-index를 사용하여 각 위치를 잡아줍니다.
코드 보기
/* mouseType */
.mouse__wrap {
width: 100%;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
color: #fff;
overflow: hidden;
flex-direction: column;
cursor: none;
}
.mouse__wrap p {
font-size: 2vw;
line-height: 2;
font-weight: 300;
}
.mouse__wrap p:last-child {
font-size: 3vw;
font-weight: 300;
}
.mouse__wrap p span {
border-bottom: 0.15vw dashed #666;
color: #b0aaff;
}
@media(max-width: 800px) {
.mouse__wrap p {
padding: 0 20px;
font-size: 20px;
line-height: 1.5;
text-align: center;
margin-bottom: 10px;
}
.mouse__wrap p:last-child {
font-size: 40px;
line-height: 1.2;
text-align: center;
word-break: keep-all;
}
}
.mouse__cursor {
position: absolute;
left: 0;
top: 0;
width: 10px;
height: 10px;
z-index: 9999;
border-radius: 50%;
background: rgba(255,255,255,0.1);
user-select: none;
pointer-events: none;
transition: transform 0.3s, opacity 0.2s;
}
.mouse__cursor2 {
position: absolute;
left: 0;
top: 0;
width: 30px;
height: 30px;
z-index: 9998;
border-radius: 50%;
background: rgba(255,255,255,0.3);
user-select: none;
pointer-events: none;
transition: transform 0.5s, opacity 0.2s;
}
.mouse__cursor.active {
transform: scale(0);
}
.mouse__cursor2.active {
transform: scale(2);
background: #3b3d6350;
}
script
이번에는 GSAP를 사용해주었으며, 마우스 오버 시에 mouseenter를 사용하여 작용하도록 했습니다.
코드 보기
const cursor = document.querySelector(".mouse__cursor");
const cursor2 = document.querySelector(".mouse__cursor2");
const span = document.querySelectorAll(".mouse__wrap span")
window.addEventListener("mousemove", e => {
// 커서 좌표값 할당
// cursor.style.left = e.pageX -5+ "px";
// cursor.style.top = e.pageY -5+ "px";
// cursor2.style.left = e.pageX -15+ "px";
// cursor2.style.top = e.pageY -15+ "px";
// GSAP
gsap.to(cursor, {duration: 0.3, left: e.pageX -5, top:e.pageY -5});
gsap.to(cursor2, {duration: 0.8, left: e.pageX -15, top:e.pageY -15});
// 오버 효과
// mouseenter / mouseover / 이벤트 버블링
span.forEach(span => {
span.addEventListener("mouseenter", () => {
cursor.classList.add("active");
cursor2.classList.add("active");
});
span.addEventListener("mouseleave", () => {
cursor.classList.remove("active");
cursor2.classList.remove("active");
});
});
});
결과물
'EFFECT' 카테고리의 다른 글
마우스 이펙트의 조명 효과를 알아보자! (0) | 2022.09.22 |
---|---|
parallax 05 (5) | 2022.09.20 |
mouse 01 (2) | 2022.09.18 |
Slider 04 (2) | 2022.09.18 |
Slider 03 (2) | 2022.09.18 |
댓글